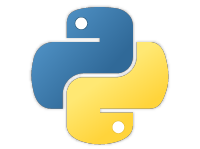
Python Introduction
Python is a general purpose high level programming language and introduced by Guido van Rossum in 1989 NRI. It is a Open source, so anyone can contribute to its development. Code that is as understandable as plain English language. It is suitable for everyday tasks, allowing for short development times.
This is becoming most demanding language why beacuse it provides very concise code and rich libraries(Batteries).
How it’s provide concise Code?
Take Java language as an example where to write a simple program we need to know what is class,package,modifiers etc.We need to write 3 to four line of coding just to print Hello Wold Program. But in Python same can be achieved in one line only.
Python Installation
Python comes with two parallel versions and not dependent on each other. Python 3 is not enhanced version of Python 2 but both are independent. It’s always recommended to start learning with Python 3 only why because 2020 onwards there is no support from Python Software foundation for Python 2.
Open www.python.org and try to download latest stable version of python.
Go to download section and download
i.e.Python 3.11.1
Environment Variable Setup
There is an option provided to set up the environment variable while Python installation time only.
i.e: Add Python 3.11 to PATH
Python installation provides below tools to execute python programs
Python REPL Tool(Python IDLE):Read Evaluate Print Loop
Package Installation Instruction and Tips
Let’s take an example to install Requests library from the Python Python Package Index(PyPI) to understand the python library installation.
Linux/macOS:
python3 -m pip install requests
Windows:
py -m pip install requests
How to install specific version of python lib?
Linux/macOS:
python3 -m pip install requests==2.18.4
Windows:
py -m pip install requests==2.18.4
How to install latest releases of python lib?
Linux/macOS:
python3 -m pip install requests>=2.0.0,<3.0.0
Windows:
py -m pip install requests>=2.0.0,<3.0.0
How to install pre-releases version of python lib?
Linux/macOS:
python3 -m pip install --pre requests
Windows:
py -m pip install --pre requests
How to upgrade installed python lib?
Linux/macOS:
python3 -m pip install --upgrade requests
Windows:
py -m pip install --upgrade requests
How to install python lib using requirements files?
Create one file named as requirements.txt as below:
cachetools==2.0.1 certifi==2017.7.27.1 chardet==3.0.4 google-auth==1.1.1 idna==2.6 pyasn1==0.3.6 pyasn1-modules==0.1.4 requests==2.18.4 rsa==3.4.2 six==1.11.0 urllib3==1.22
Linux/macOS:
python3 -m pip install -r requirements.txt
Windows:
py -m pip install -r requirements.txt
How to list down the installed python library?
Linux/macOS:
python3 -m pip freeze
Windows:
py -m pip freeze
Python Basics
Reserved Keywords
There are 33 reserved words are available in Python.
True,False,None
and,or,not,is
if,else,elif
while,for,break,continue,return,in,yield
try,except,finally,raise(To throw custom exceptions similar like java throw),assert(For debugging purposes)
import,from,as(To create alias),class,def(To declare method),pass(Not going to do anything)
global(To declare global variable),nonlocal(To declare local variable),lambda(To create anonymous funtions)
del(To delete a variable or method or class),with(In File handling will be used)
Note:
1)All reserved words contain only alphabets.
2)Except first 3 contain lower case alphabets.
How to check reserved keywords in Python using Python Program?
>>> import keyword
>>> keyword.kwlist
[‘False’, ‘None’, ‘True’, ‘and’, ‘as’, ‘assert’, ‘async’, ‘await’, ‘break’,
‘class’, ‘continue’, ‘def’, ‘del’, ‘elif’, ‘else’, ‘except’, ‘finally’, ‘for’,
‘from’, ‘global’, ‘if’, ‘import’, ‘in’, ‘is’, ‘lambda’, ‘nonlocal’, ‘not’, ‘or’,
‘pass’, ‘raise’, ‘return’, ‘try’, ‘while’, ‘with’, ‘yield’]
Python Data Types
Data Types
Since python is a dynamically typed programming language so no need to define datatype manually instead it will be identified based on value data type implicitly.
Data Type is a Type of value represented by the variable.
Various Data Types defined in Python as below:
1)int
2)float
3)complex
4)bool
5)str
6)bytes
7)bytearray
8)range
9)list
10)tuple
11)set
12)frozenset
13)dict
14)None
Note: In Python every thing is an object even an method also.
Explanation about each Data type:
1) int Data Type:
Python uses int data type to represent Integral values.
Program:
>>> a=10
>>> type(a)
Output:class ‘int’
In how many ways we can represent int type:By default Python considers as decimal form only.
1)Decimal Form(Base-10):0 to 9
2)Binary Form(Base-2):0 and 1
Program
a=0b1111
a=0B1111
Output:15
Program:
a=-0b1111
output:-15
3)Octal Form(Base-8):0 to 7
Program:
>>> a=0o777
>>> a
Output:511
Program:
>>> a=0O777
>>> a
Output:511
4)Hexa Decimal(Base-16):0 to 9,a to f,A to F(Here Python is not case sensitive)
Program:
>>> a=0XFace
>>> a
Output:64206
Program:
>>> a=0xBeef
>>> a
Output:48879
Program:
>>> a=0xBeer
Output:SyntaxError: invalid syntax
2) float Data Type
Program:
>>> f=123.4435
>>>
>>> f
123.4435
Note:Exponential form is allowed under float data type.Big benefit of defining into exponential form is any bigger value can be represented into a shorter way.
Program:
>>> x=1.2e3
>>> x
1200.0
3) complex Data Type
If you want to develop scientific/complex program then always recommend to go for complex data type.
Syntax for complex data type is as a+bj
Where a represents Real Part and can be any data type
b represents Imaginary Part and can be only decimal.
j^2=-1 where j is mandatory.
Program:
>>> x=10+20j
>>> x
(10+20j)
>>> type(x)
Program:
>>> p=0b111+10j
>>> p
(7+10j)
Program: Operations with two complex equations
>>> a=10+20j
>>> b=20+10j
>>> a+b
(30+30j)
>>> a-b
(-10+10j)
To know about real and imaginary part using program
>>>a=10+20j
>>> a.real Here real is pre defined attribute/property by Python
10.0
>>> a.imag Here imag is pre defined attribute/property by Python
20.0
4) bool Data Type
Allowed values are True and False for bool data type where T and F always should be in caps.
Program:
>>> a=True
>>> a
True
>>> type(a)
>>> a=true
Traceback (most recent call last):
File “”, line 1, in
a=true
NameError: name ‘true’ is not defined
Program:
>>> a=’true’
>>> a
‘true’
>>> type(a)
Program:
>>> True+True
2
>>> False+False
0
>>> True+False
1
5) str Data type
Any sequence of character is know as String.
Here we can represent str by using single quote or double quote but single quote is recommended.
Program:
>>> s=pradeep
Traceback (most recent call last):
File “”, line 1, in
s=pradeep
NameError: name ‘pradeep’ is not defined
>>> s=’pradeep’
>>> s
‘pradeep’
>>> s=”pradeep”
>>> s
‘pradeep’
Note:For multiple line string literals we always need to use triple single/double quote.
Program:
If we use single quote to print multiple line string literals.
Test.py
s=’pradeep
singh’
print(s)
Result:
D:\Python\Programs>py Test.py
File “Test.py”, line 1
s=’pradeep
^
SyntaxError: EOL while scanning string literal
Use triple single quote
Test.py
s=”’pradeep
singh”’
print(s)
Result:
D:\Python\DurgaSir\Programs>py Test.py
pradeep
singh
slice Operator
To get a sub string(piece) of a string you need to use slice operator.
Syntax: s[begin:end]=>Returns substring from begin index to end-1 index.
Program:
>>> s=”I am a software engineer”
>>> s[2:5]
‘am ‘
>>> s[1:10]
‘ am a sof’
>>> s[0:10]
‘I am a sof’
Default value for end index is end of the string if end index is not provided.
Program:
>>> s=’pradeep’
>>> s[2:]
‘adeep’
Default value for begin index is Start from the string if begin index is not provided.
Program:
>>> s=’pradeep’
>>> s[:3]
‘pra’
If not begin and end index is provided
Program:
>>> s=’pradeep’
>>> s[:]
‘pradeep’
How can we achieve jump by steps while getting sub string.
Syntax: s[begin:end:step]
Program:
>>> s=’iamasoftwareengineer’
>>> s[1:10:2]
‘aaota’
>>> s[1:10:3]
‘ast’
Note: Python supports positive(Left to right) and negative(Right to Left) index both.
Program:
>>> s=’pradeep’
>>> s[0]
‘p’
>>> s[1]
‘r’
>>> s[2]
‘a’
>>> s[3]
‘d’
>>> s[4]
‘e’
>>> s[-1]
‘p’
>>> s[-2]
‘e’
>>> s[-3]
‘e’
Repetition Operator:
Syntax: s*n->Repeats s String to n times
Program:
>>> s=’pradeep’
>>> s*3
‘pradeeppradeeppradeep’
>>> s*10
‘pradeeppradeeppradeeppradeeppradeeppradeeppradeeppradeeppradeeppradeep’
Length of string:
Program:
>>> s=’pradeep’
>>> len(s)
7
Python’s Fundamental datatypes:
1)char ==>str type only
2)long ==>int type only
Type Casting or Type coersion:Python supports data type casting using multiple inbuilt functions
1)int():To convert any other type value to int type.
Program:
>>> int(123.345)
123
Note:Can not convert complex type to int type
Program:
>>> int(10+20j)
Traceback (most recent call last):
File “”, line 1, in
int(10+20j)
TypeError: can’t convert complex to int
Boolean to int:
>>> int(True)
1
>>> int(False)
0
str to int:String should contain only base 10 value.
Program:
>>> int(’10’)
10
Program:
>>> int(“10.5”)
Traceback (most recent call last):
File “”, line 1, in
int(“10.5”)
ValueError: invalid literal for int() with base 10: ‘10.5’
Program:
>>> int(0b1111)
15
>>> int(‘0b1111’)
Traceback (most recent call last):
File “”, line 1, in
int(‘0b1111’)
ValueError: invalid literal for int() with base 10: ‘0b1111’
2)float()
Program:
>>> float(10)
10.0
Program:
>>> float(10+20j)
Traceback (most recent call last):
File “”, line 1, in
float(10+20j)
TypeError: can’t convert complex to float
Program:
>>> float(True)
1.0
>>> float(False)
0.0
Program:
>>> float(’10’)
10.0
>>> float(‘10.5’)
10.5
Program:
>>> float(‘ten’)
Traceback (most recent call last):
File “”, line 1, in
float(‘ten’)
ValueError: could not convert string to float: ‘ten’
Function:complex()
Will be used to conver other types to complex type.
There are two forms of complex function found:
1)Form1: complex(x)=>Its implies that x+0j
2)Form 2: complex(x,y)=>x+yj
Programs:
>>> complex(10)
(10+0j)
>>> complex(10,20)
(10+20j)
>>> complex(True)
(1+0j)
>>> complex(True,True)
(1+1j)
>>> complex(False)
0j
>>> complex(False,False)
0j
>>> complex(False,True)
1j
>>> complex(“10”)
(10+0j)
>>> complex(“10.5”)
(10.5+0j)
>>> complex(“ten”)
Traceback (most recent call last):
File “<pyshell#9>”, line 1, in <module>
complex(“ten”)
ValueError: complex() arg is a malformed string
>>> complex(0B1111)
(15+0j)
>>> complex(“5″,”10”)>>> complex(“5″,”10”) Traceback (most recent call last): File “<pyshell#12>”, line 1, in <module> complex(“5″,”10”)TypeError: complex() can’t take second arg if first is a string
Function: bool()
To convert other types to boolean.
Int Argument:
If we pass non zero value then bool returns always true other wise false.
Program:
>>> bool(1)
True
>>> bool(10)
True
>>> bool(0)
False
>>> bool(12)
True
float Argument:
If we pass except 0.0 value then bool returns always true.
Program:
>>> bool(0.0)
False
>>> bool(0.1)
True
complex Argument:
If both real and imaginary part are 0 then bool returns false other wise true.
Program:
>>> bool(10+20j)
True
>>> bool(0+10j)
True
>>> bool(0+0j)
False
>>> bool(0j)
False
>>> bool(1j)
str Argument:
if argument is empty string then bool returs as false other wise returns true.
>>> bool(”)
False
>>> bool(“pradeep”)
True
>>> bool(‘ ‘)
True
Note:bool function never throws error for any data types.